Enrollment options
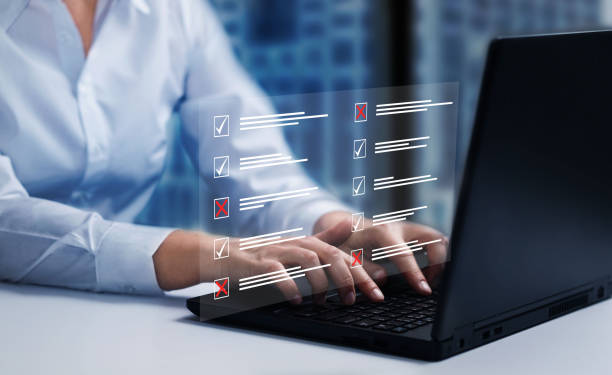
This self-paced course is made up of modules that will help you build your understanding of LoRa® and LoRaWAN® from the ground up. Each module has clear Learning Objectives, and the quizzes at the end of each module will help ensure that you have mastered the material.
In addition to the core coursework, most modules offer Advanced Assignments that include additional reading material and hands-on practice.
- Teacher: Semtech .